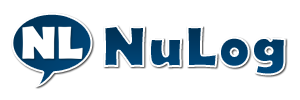
Getting Started (Quick Start)¶
If you are totally new to logging in software, first read Introduction to Logging.
If you are new to tag-based logging, read Introduction to Tag-Based Logging.
Step 1: Install Using NuGet¶
NuLog is delivered using NuGet. Look for, and install the NuLog package into any project you wish to use NuLog for. NuLog supports .Net versions 3.5, 4 and 4.5.2 (and on).
You’ll want to get your configuration squared away. For more information on configuring NuLog, head over to Configuration Template.
Step 2: Get Standard Logger¶
1 2 3 4 5 6 7 8 9 10 11 | // use NuLog
using NuLog;
namespace NuLogSnippets.Docs {
public class GetStandardLogger {
// Get the logger from the log manager
private static readonly ILogger _logger = LogManager.GetLogger();
}
}
|
Step 3: Log¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | using NuLog;
namespace NuLogSnippets.Docs {
public class GetStandardLogger {
private static readonly ILogger _logger = LogManager.GetLogger();
public void DoSomething() {
// Log something
_logger.Log("Hello, world! I'll be dispatched later.");
_logger.LogNow("Hello, world! I'll be dispatched immediately.");
}
}
}
|
Log vs. LogNow¶
NuLog is designed to defer the actual action of dispatching and logging a log event to a queue that is managed by a background process (thread). This allows control to return to the logging method much sooner, instead of having to wait for the log message to be dispatched before returning. When calling Log
on the Logger, the log event is generated by the Logger, then added to a queue for dispatch by a background process. To dispatch a log event for immediate logging, use LogNow
instead.